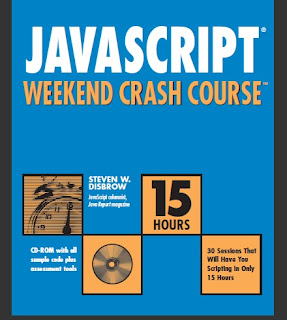
1. Where can you use the <script> </script> tags in HTML file?
2. What is a function?
3. What are the possible values for a variable that contains a Boolean value?
4. In your own words, describe what a code block is.
5. What rules must a JavaScript variable and function name follow?
6. What are the two ways to include comments in your JavScript source code?
7. What file format should your JavaScript code be stored in?
8. What is the language attribute of the <script> tag used for?
9. How do you return a value from a function?
10. Write a function that takes four Boolean parameters and returns a true only if all four parameters are true.
11. Write a loop that adds the numbers from 1 to 100 and prints the result.
12. How many parameters must a function have?
13. What is the src attribute of the <script> tag used for?
14. In your own words, describe what the three expressions in the condition of a for loop do.
15. What is the difference between local and global variables?
16. What a value will be played in x as a result of the following statements?
What will the value of y be?
y - 75:
x - (y - 100) ? 29 : 42 :
17. What kind of data can you assign to a JavaScript variable?
18. Create a Ffunction to square a number and return the result.
19. Create a function that takes one number as a parameter.
If the number is odd, the function should return true, otherwise, it should return false
20. Write a function that accepts a number as a parameter and return the boolean equivalent for that number.
Download this tutorial in Here PDF | 3,60 MB
Advanced Java 2
Platform
HOW TO PROGRAM
Preface xxi
1 Introduction
1.1 Introduction
1.2 Architecture of the Book
1.2.1 Advanced GUI, Graphics and JavaBeans
1.2.2 Distributed Systems
1.2.3 Web Services
1.2.4 Enterprise Java
1.2.5 Enterprise Case Study
1.2.6 XML
1.3 Tour of the Book
1.4 Running Example Code
1.5 Design Patterns
1.5.1 History of Object-Oriented Design Patterns
1.5.2 Design Patterns Discussion
1.5.3 Concurrency Patterns
1.5.4 Architectural Patterns
1.5.5 Further Study on Design Patterns
2 Advanced Swing Graphical User Interface
Components
2.1 Introduction
2.2 WebBrowser Using JEditorPane and JToolBar
2.2.1 Swing Text Components and HTML Rendering
2.2.2 Swing Toolbars
2.3 Swing Actions
2.4 JSplitPane and JTabbedPane
2.5 Multiple-Document Interfaces
2.6 Drag and Drop
2.7 Internationalization
2.8 Accessibility
2.9 Internet and World Wide Web Resources
3 Model-View-Controller
3.1 Introduction
3.2 Model-View-Controller Architecture
3.3 Observable Class and Observer Interface
3.4 JList
3.5 JTable
3.6 JTree
3.6.1 Using DefaultTreeModel
3.6.2 Custom TreeModel Implementation
4 Graphics Programming with Java 2D and Java 3D
4.1 Introduction
4.2 Coordinates, Graphics Contexts and Graphics Objects
4.3 Java 2D API
4.3.1 Java 2D Shapes
4.3.2 Java 2D Image Processing
4.4 Java 3D API
4.4.1 Obtaining and Installing the Java 3D API
4.4.2 Java 3D Scenes
4.4.3 A Java 3D Example
4.5 A Java 3D Case Study: A 3D Game with Custom Behaviors
5 Case Study: Java 2D GUI Application with
Design Patterns
5.1 Introduction
5.2 Application Overview
5.3 MyShape Class Hierarchy
5.4 Deitel DrawingModel
5.5 Deitel Drawing Views
5.6 Deitel Drawing Controller Logic
5.6.1 MyShapeControllers for Processing User Input
5.6.2 MyShapeControllers and Factory Method Design Pattern
5.6.3 Drag-and-Drop Controller
5.7 DrawingInternalFrame Component
5.8 ZoomDialog, Action and Icon Components
5.9 DeitelDrawing Application
6 JavaBeans Component Model
6.1 Introduction
6.2 Using Beans in Forte for Java Community Edition
6.3 Preparing a Class to be a JavaBean
6.4 Creating a JavaBean: Java Archive Files
6.5 JavaBean Properties
6.6 Bound Properties
6.7 Indexed Properties and Custom Events 355
6.8 Customizing JavaBeans for Builder Tools 364
6.8.1 PropertyEditors 371
6.8.2 Customizers 375
6.9 Internet and World Wide Web Resources
7 Security
7.1 Introduction
7.2 Ancient Ciphers to Modern Cryptosystems
7.3 Secret-Key Cryptography
7.4 Public-Key Cryptography
7.5 Cryptanalysis
7.6 Key Agreement Protocols
7.7 Key Management
7.8 Java Cryptography Extension (JCE)
7.8.1 Password-Based Encoding with JCE
7.8.2 Decorator Design Pattern
7.9 Digital Signatures
7.10 Public-Key Infrastructure, Certificates and Certification Authorities
7.10.1 Java Keystores and keytool
7.11 Java Policy Files
7.12 Digital Signatures for Java Code
7.13 Authentication
7.13.1 Kerberos
7.13.2 Single Sign-On
7.13.3 Java Authentication and Authorization Service (JAAS)
7.14 Secure Sockets Layer (SSL)
7.14.1 Java Secure Socket Extension (JSSE)
7.15 Java Language Security and Secure Coding
7.16 Internet and World Wide Web Resources
8 Java Database Connectivity (JDBC)
8.1 Introduction
8.2 Relational-Database Model
8.3 Relational Database Overview: The books Database
8.4 Structured Query Language (SQL)
8.4.1 Basic SELECT Query
8.4.2 WHERE Clause
8.4.3 ORDER BY Clause
8.4.4 Merging Data from Multiple Tables: Joining
8.4.5 INSERT INTO Statement
8.4.6 UPDATE Statement
8.4.7 DELETE FROM Statement
8.5 Creating Database books in Cloudscape
8.6 Manipulating Databases with JDBC
8.6.1 Connecting to and Querying a JDBC Data Source
8.6.2 Querying the books Database
8.7 Case Study: Address-Book Application
8.7.1 PreparedStatements
8.7.2 Transaction Processing
8.7.3 Address-Book Application
8.8 Stored Procedures
8.9 Batch Processing
8.10 Processing Multiple ResultSets or Update Counts
8.11 Updatable ResultSets
8.12 JDBC 2.0 Optional Package javax.sql
8.12.1 DataSource
8.12.2 Connection Pooling
8.12.3 RowSets
8.13 Internet and World Wide Web Resources
9 Servlets
9.1 Introduction
9.2 Servlet Overview and Architecture
9.2.1 Interface Servlet and the Servlet Life Cycle
9.2.2 HttpServlet Class
9.2.3 HttpServletRequest Interface
9.2.4 HttpServletResponse Interface
9.3 Handling HTTP get Requests
9.3.1 Setting Up the Apache Tomcat Server
9.3.2 Deploying a Web Application
9.4 Handling HTTP get Requests Containing Data
9.5 Handling HTTP post Requests
9.6 Redirecting Requests to Other Resources
9.7 Session Tracking
9.7.1 Cookies
9.7.2 Session Tracking with HttpSession
9.8 Multi-Tier Applications: Using JDBC from a Servlet
9.9 HttpUtils Class
9.10 Internet and World Wide Web Resources
10 JavaServer Pages (JSP)
10.1 Introduction
10.2 JavaServer Pages Overview
10.3 A First JavaServer Page Example
10.4 Implicit Objects
10.5 Scripting
10.5.1 Scripting Components
10.5.2 Scripting Example
10.6 Standard Actions
10.6.1 <jsp:include> Action
10.6.2 <jsp:forward> Action
10.6.3 <jsp:plugin> Action
10.6.4 <jsp:useBean> Action
10.7 Directives
10.7.1 page Directive
10.7.2 include Directive
10.8 Custom Tag Libraries
10.8.1 Simple Custom Tag
10.8.2 Custom Tag with Attributes
10.8.3 Evaluating the Body of a Custom Tag
10.9 Internet and World Wide Web Resources
11 Case Study: Servlet and JSP Bookstore
11.1 Introduction
11.2 Bookstore Architecture
11.3 Entering the Bookstore
11.4 Obtaining the Book List from the Database
11.5 Viewing a Book’s Details
11.6 Adding an Item to the Shopping Cart
11.7 Viewing the Shopping Cart
11.8 Checking Out
11.9 Processing the Order
11.10 Deploying the Bookstore Application in J2EE 1.2.1
11.10.1 Configuring the books Data Source
11.10.2 Launching the Cloudscape Database and J2EE Servers
11.10.3 Launching the J2EE Application Deployment Tool
11.10.4 Creating the Bookstore Application
11.10.5 Creating BookServlet and AddToCartServlet Web Components
11.10.6 Adding Non-Servlet Components to the Application
11.10.7 Specifying the Web Context, Resource References, JNDI
Names and Welcome Files
11.10.8 Deploying and Executing the Application
12 Java-Based Wireless Applications
Development and J2ME
12.1 Introduction
12.2 WelcomeServlet Overview
12.3 TipTestServlet Overview
12.3.1 Internet Explorer Request
12.3.2 WAP Request
12.3.3 Pixo i-mode Request
12.3.4 J2ME Client Request
12.4 Java 2 Micro Edition
12.4.1 Connected Limited Device Configuration (CLDC)
12.4.2 Mobile Information Device Profile (MIDP)
12.4.3 TipTestMIDlet Overview
12.5 Installation Instructions
12.6 Internet and World Wide Web Resources
13 Remote Method Invocation
13.1 Introduction
13.2 Case Study: Creating a Distributed System with RMI
13.3 Defining the Remote Interface
13.4 Implementing the Remote Interface
13.5 Compiling and Executing the Server and the Client
13.6 Case Study: Deitel Messenger with Activatable Server
13.6.1 Activatable Deitel Messenger ChatServer
13.6.2 Deitel Messenger Client Architecture and Implementation
13.6.3 Running the Deitel Messenger Server and Client Applications
13.7 Internet and World Wide Web Resources
14 Session EJBs and Distributed Transactions
14.1 Introduction
14.2 EJB Overview
14.2.1 Remote Interface
14.2.2 Home Interface
14.2.3 EJB Implementation
14.2.4 EJB Container
14.3 Session Beans
14.3.1 Stateful Session EJBs
14.3.2 Deploying Session EJBs
14.3.3 Stateless Session EJBs
14.4 EJB Transactions
14.4.1 MoneyTransfer EJB Home and Remote Interfaces
14.4.2 Bean-Managed Transaction Demarcation
14.4.3 Container-Managed Transaction Demarcation
14.4.4 MoneyTransfer EJB Client
14.4.5 Deploying the MoneyTransfer EJB
14.5 Internet and World Wide Web Resources
15 Entity EJBs
15.1 Introduction
15.2 Entity EJB Overview
15.3 Employee Entity EJB
15.4 Employee EJB Home and Remote Interfaces
15.5 Employee EJB with Bean-Managed Persistence
15.5.1 Employee EJB Implementation
15.5.2 Employee EJB Deployment
15.6 Employee EJB with Container-Managed Persistence
15.7 Employee EJB Client
15.8 Internet and World Wide Web Resources
16 Messaging with JMS
16.1 Introduction
16.2 Installation and Configuration of J2EE 1.3
16.3 Point-To-Point Messaging
16.3.1 Voter Application: Overview
16.3.2 Voter Application: Sender Side
16.3.3 Voter Application: Receiver Side
16.3.4 Voter Application: Configuring and Running
16.4 Publish/Subscribe Messaging
16.4.1 Weather Application: Overview
16.4.2 Weather Application: Publisher Side
16.4.3 Weather Application: Subscriber Side
16.4.4 Weather Application: Configuring and Running
16.5 Message-Driven Enterprise JavaBeans
16.5.1 Voter Application: Overview
16.5.2 Voter Application: Receiver Side
16.5.3 Voter Application: Configuring and Running
17 Enterprise Java Case Study: Architectural Overview
17.1 Introduction
17.2 Deitel Bookstore
17.3 System Architecture
17.4 Enterprise JavaBeans
17.4.1 Entity EJBs
17.4.2 Stateful Session EJBs
17.5 Servlet Controller Logic
17.6 XSLT Presentation Logic
18 Enterprise Java Case Study: Presentation
and Controller Logic
18.1 Introduction
18.2 XMLServlet Base Class
18.3 Shopping Cart Servlets
18.3.1 AddToCartServlet
18.3.2 ViewCartServlet
18.3.3 RemoveFromCartServlet
18.3.4 UpdateCartServlet
18.3.5 CheckoutServlet
18.4 Product Catalog Servlets
18.4.1 GetAllProductsServlet
18.4.2 GetProductServlet
18.4.3 ProductSearchServlet
18.5 Customer Management Servlets
18.5.1 RegisterServlet
18.5.2 LoginServlet
18.5.3 ViewOrderHistoryServlet
18.5.4 ViewOrderServlet
18.5.5 GetPasswordHintServlet
19 Enterprise Java Case Study: Business Logic Part 1
19.1 Introduction
19.2 EJB Architecture
19.3 ShoppingCart Implementation
19.3.1 ShoppingCart Remote Interface
19.3.2 ShoppingCartEJB Implementation
19.3.3 ShoppingCartHome Interface
19.4 Product Implementation
19.4.1 Product Remote Interface
19.4.2 ProductEJB Implementation
19.4.3 ProductHome Interface
19.4.4 ProductModel
19.5 Order Implementation
19.5.1 Order Remote Interface
19.5.2 OrderEJB Implementation
19.5.3 OrderHome Interface
19.5.4 OrderModel
19.6 OrderProduct Implementation
19.6.1 OrderProduct Remote Interface
19.6.2 OrderProductEJB Implementation
19.6.3 OrderProductHome Interface
19.6.4 OrderProductPK Primary-Key Class
19.6.5 OrderProductModel
20 Enterprise Java Case Study: Business Logic Part 2
20.1 Introduction
20.2 Customer Implementation
20.2.1 Customer Remote Interface
20.2.2 CustomerEJB Implementation
20.2.3 CustomerHome Interface
20.2.4 CustomerModel
20.3 Address Implementation
20.3.1 Address Remote Interface
20.3.2 AddressEJB Implementation
20.3.3 AddressHome Interface
20.3.4 AddressModel
20.4 SequenceFactory Implementation
20.4.1 SequenceFactory Remote Interface
20.4.2 SequenceFactoryEJB Implementation
20.4.3 SequenceFactoryHome Interface
20.5 Deitel Bookstore Application Deployment with J2EE
20.5.1 Deploying Deitel Bookstore CMP Entity EJBs
20.5.2 Deploying Deitel Bookstore Servlets
21 Application Servers
21.1 Introduction
21.2 J2EE Specification and Benefits
21.3 Commercial Application Servers
21.3.1 BEA WebLogic 6.0
21.3.2 iPlanet Application Server 6.0
21.3.3 IBM WebSphere Advanced Application Server 4.0
21.3.4 JBoss 2.2.2 Application Server
21.4 Deploying the Deitel Bookstore on BEA WebLogic
21.5 Deploying the Deitel Bookstore on IBM WebSphere
21.6 Internet and World Wide Web Resources
22 Jini
22.1 Introduction
22.2 Installing Jini
22.3 Configuring the Jini Runtime Environment
22.4 Starting the Required Services
22.5 Running the Jini LookupBrowser
22.6 Discovery
22.6.1 Unicast Discovery
22.6.2 Multicast Discovery
22.7 Jini Service and Client Implementations
22.7.1 Service Interfaces and Supporting Classes
22.7.2 Service Proxy and Service Implementations
22.7.3 Registering the Service with Lookup Services
22.7.4 Jini Service Client
22.8 Introduction to High-Level Helper Utilities
22.8.1 Discovery Utilities
22.8.2 Entry Utilities
22.8.3 Lease Utilities
22.8.4 JoinManager Utility
22.8.5 Service Discovery Utilities
22.9 Internet and World Wide Web Resources
23 JavaSpaces
23.1 Introduction
23.2 JavaSpaces Service Properties
23.3 JavaSpaces Service
23.4 Discovering the JavaSpaces Service
23.5 JavaSpace Interface
23.6 Defining an Entry
23.7 Write Operation
23.8 Read and Take Operations
23.8.1 Read Operation
23.8.2 Take Operation
23.9 Notify Operation
23.10 Method snapshot
23.11 Updating Entries with Jini Transaction Service
23.11.1 Defining the User Interface
23.11.2 Discovering the TransactionManager Service
23.11.3 Updating an Entry
23.12 Case Study: Distributed Image Processing
23.12.1 Defining an Image Processor
23.12.2 Partitioning an Image into Smaller Pieces
23.12.3 Compiling and Running the Example
23.13 Internet and World Wide Web Resources
24 Java Management Extensions (JMX) (on CD)
24.1 Introduction
24.2 Installation
24.3 Case Study
24.3.1 Instrument Resources
24.3.2 Implementation of the JMX Management Agent
24.3.3 Broadcasting and Receiving Notifications
24.3.4 Management Application
24.3.5 Compiling and Running the Example
24.4 Internet and World Wide Web Resources
25 Jiro (on CD)
25.1 Introduction
25.2 Installation
25.3 Starting Jiro
25.4 Dynamic vs. Static Services
25.5 Dynamic Services
25.5.1 Dynamic-Service Implementation
25.6 Static Services
25.6.1 Locating Static Services with Class ServiceFinder
25.6.2 Event Service
25.6.3 Log Service
25.6.4 Scheduling Service
25.7 Dynamic Service Deployment
25.7.1 Dynamic–Service Usage
25.8 Management Policies
25.8.1 Policy–Management Deployment
25.9 Closing Notes on the Printer Management Solution
25.10 Internet and World Wide Web Resources
26 Common Object Request Broker Architecture
(CORBA): Part 1 (on CD)
26.1 Introduction
26.2 Step-by-Step
26.3 First Example: SystemClock
26.3.1 SystemClock.idl
26.3.2 SystemClockImpl.java
26.3.3 SystemClockClient.java
26.3.4 Running the Example
26.4 Technical/Architectural Overview
26.5 CORBA Basics
26.6 Example: AlarmClock
26.6.1 AlarmClock.idl
26.6.2 AlarmClockImpl.java
26.6.3 AlarmClockClient.java
26.7 Distributed Exceptions
26.8 Case Study: Chat
26.8.1 chat.idl
26.8.2 ChatServerImpl.java
26.8.3 DeitelMessenger.java
26.8.4 Running Chat
26.8.5 Issues
26.9 Comments and Comparisons
26.10 Internet and World Wide Web Resources
27 Common Object Request Broker Architecture
(CORBA): Part 2 (on CD)
27.1 Introduction
27.2 Static Invocation Interface (SII), Dynamic Invocation Interface (DII)
and Dynamic Skeleton Interface (DSI)
27.3 BOAs, POAs and TIEs
27.4 CORBAservices
27.4.1 Naming Service
27.4.2 Security Service
27.4.3 Object Transaction Service
27.4.4 Persistent State Service
27.4.5 Event and Notification Services
27.5 EJBs and CORBAcomponents
27.6 CORBA vs. RMI
27.6.1 When to Use RMI
27.6.2 When to Use CORBA
27.6.3 RMI-IIOP
27.7 RMIMessenger Case Study Ported to RMI-IIOP
27.7.1 ChatServer RMI-IIOP Implementation
27.7.2 ChatClient RMI-IIOP Implementation
27.7.3 Compiling and Running the ChatServer and ChatClient
27.8 Future Directions
27.9 Internet and World Wide Web Resources
28 Peer-to-Peer Applications and JXTA
28.1 Introduction
28.2 Client/Server and Peer-to-Peer Applications
28.3 Centralized vs. Decentralized Network Applications
28.4 Peer Discovery and Searching
28.5 Case Study: Deitel Instant Messenger
28.6 Defining the Service Interface
28.7 Defining the Service implementation
28.8 Registering the Service
28.9 Find Other Peers
28.10 Compiling and Running the Example
28.11 Improving Deitel Instant Messenger
28.12 Deitel Instant Messenger with Multicast Sockets
28.12.1 Registering the Peer
28.12.2 Finding Other Peers
28.13 Introduction to JXTA
28.14 Internet and World Wide Web Resources
29 Introduction to Web Services and SOAP
29.1 Introduction
29.2 Simple Object Access Protocol (SOAP)
29.3 SOAP Weather Service
29.4 Internet and World Wide Web Resources
A Creating Markup with XML (on CD)
A.1 Introduction
A.2 Introduction to XML Markup
A.3 Parsers and Well-Formed XML Documents
A.4 Characters
A.4.1 Characters vs. Markup
A.4.2 White Space, Entity References and Built-In Entities
A.5 CDATA Sections and Processing Instructions
A.6 XML Namespaces
A.7 Internet and World Wide Web Resources
B Document Type Definition (DTD) (on CD)
B.1 Introduction
B.2 Parsers, Well-Formed and Valid XML Documents
B.3 Document Type Declaration
B.4 Element Type Declarations
B.4.1 Sequences, Pipe Characters and Occurrence Indicators
B.4.2 EMPTY, Mixed Content and ANY
B.5 Attribute Declarations
B.6 Attribute Types
B.6.1 Tokenized Attribute Type (ID, IDREF, ENTITY, NMTOKEN)
B.6.2 Enumerated Attribute Types
B.7 Conditional Sections
B.8 Whitespace Characters
B.9 Internet and World Wide Web Resources
C Document Object Model (DOM™) (on CD)
C.1 Introduction
C.2 DOM with Java
C.3 Setup Instructions
C.4 DOM Components
C.5 Creating Nodes
C.6 Traversing the DOM
C.7 Internet and World Wide Web Resources
D XSL: Extensible Stylesheet Language
Transformations (XSLT) (on CD)
D.1 Introduction
D.2 Applying XSLTs with Java
D.3 Templates
D.4 Creating Elements and Attributes
D.5 Iteration and Sorting
D.6 Conditional Processing
D.7 Combining Style Sheets
D.8 Variables
D.9 Internet and World Wide Web Resources
E Downloading and Installing J2EE 1.2.1 (on CD)
E.1 Introduction
E.2 Installation
E.3 Configuration
E.3.1 JDBC Drivers and Data Sources
E.3.2 HTTP properties
F Java Community ProcessSM (JCP) (on CD)
F.1 Introduction
F.2 Participants
F.2.1 Program Management Office
F.2.2 Executive Committee
F.2.3 Experts
F.2.4 Members
F.2.5 Public Participation
F.3 Java Community Process
F.3.1 Initiation Phase
F.3.2 Community Draft Phase
F.3.3 Public Draft Phase
F.3.4 Final Phase
F.3.5 Maintenance Phase
G Java Native Interface (JNI) (on CD)
G.1 Introduction
G.2 Getting Started with Java Native Interface
G.3 Accessing Java Methods and Objects from Native Code
G.4 JNI and Arrays
G.5 Handling Exceptions with JNI
G.6 Internet and World Wide Web Resources
H Career Opportunities (on CD)
H.1 Introduction
H.2 Resources for the Job Seeker
H.3 Online Opportunities for Employers
H.3.1 Posting Jobs Online
H.3.2 Problems with Recruiting on the Web
H.3.3 Diversity in the Workplace
H.4 Recruiting Services
H.4.1 Testing Potential Employees Online
H.5 Career Sites
H.5.1 Comprehensive Career Sites
H.5.2 Technical Positions
H.5.3 Wireless Positions
H.5.4 Contracting Online
H.5.5 Executive Positions
H.5.6 Students and Young Professionals
H.5.7 Other Online Career Services
H.6 Internet and World Wide Web Resources
I Unicode® (on CD)
I.1 Introduction
I.2 Unicode Transformation Formats
I.3 Characters and Glyphs
I.4 Advantages/Disadvantages of Unicode
I.5 Unicode Consortium’s Web Site
I.6 Using Unicode
I.7 Character Ranges
Index
Please download here PDF | 17.76 MB
Java Script Reference Guide
Table of Contents
1. Introduction to JavaScript
• What is JavaScript
• Uses of JavaScript
• Writing JavaScirpt
2. Programming Basics
• Variables
• Functions
3. JavaScript Objects
• Objects
• Properties
• Methods
4. Document and Window Objects
• Write to a Document
• Open a Window
5. JavaScript Events
• Events and Objects
• Image Rollovers
Download here PDF | 361,64 MB
Javascript for beginners
Table of contents
The Basics
The Necessary Software
HTML
What are HTML Pages?
Brief HTML Reference Guide
HTML and JavaScript
Incorporation in the Header
Carrying out Code Given Particular Actions
Incorporation in the Body
First JavaScript Programming
Hello World
Hello World without Parameters
Hello World with Parameters
What time is it?
Page Reference
Event Handler
onLoad
onUnload
onMouseOver
onMouseOut
onFocus
onBlur
onChange
onClick
javascript
onSubmit
Functions
Variables
Local Variables
Global Variables
Mathematical Operations
Repeated Performance
Looping with for
Looping with while
Conditional Operations
Standard Objects
document
Colors in the Document
Document Properties
Pictures in a Document
document.frames
document.forms
Text Entry Fields
Radio and Check buttons
Drop-Down Lists
Pizza Service
Euro Calculator
Strings
The String Object
length
substring
toLowerCase
toUpperCase
Moving Text
User-Defined Objects
Arrays
Working with Frames
Quiz
The Explorer
The Project
The Practice
The Main Page
The Content Page
The Explorer Page
Customization
Reserved Words
The Last Word
Please download here
JavaScript & jQuery: The Missing Manual, Second Edition
Table of contents
The Missing Credits
Introduction.
Part One: Getting Started with JavaScript
Chapter 1: Writing Your First JavaScript Program
Introducing Programming
What’s a Computer Program?
How to Add JavaScript to a Page
External JavaScript Files
Your First JavaScript Program
Writing Text on a Web Page
Attaching an External JavaScript File
Tracking Down Errors
The Firefox JavaScript Console
Displaying the Internet Explorer 9 Console
Opening the Chrome JavaScript Console
Accessing the Safari Error Console
Chapter 2: The Grammar of JavaScript
Statements
Built-In Functions
Types of Data
Numbers
Strings
Booleans
Variables
Creating a Variable
Using Variables
Working with Data Types and Variables
Basic Math.
The Order of Operations
Combining Strings
Combining Numbers and Strings
Changing the Values in Variables
Tutorial: Using Variables to Create Messages
Tutorial: Asking for Information
Arrays
Creating an Array
Accessing Items in an Array
Adding Items to an Array
Deleting Items from an Array
Tutorial: Writing to a Web Page Using Arrays
A Quick Object Lesson
Comments
When to Use Comments
Comments in This Book
Chapter 3: Adding Logic and Control to Your Programs
Making Programs React Intelligently
Conditional Statement Basics.
Adding a Backup Plan
Testing More Than One Condition
More Complex Conditions
Nesting Conditional Statements
Tips for Writing Conditional Statements
Tutorial: Using Conditional Statements
Handling Repetitive Tasks with Loops
While Loops
Loops and Arrays.
For Loops
Do/While Loops.
Functions: Turn Useful Code Into Reusable Commands
Mini-Tutorial
Giving Information to Your Functions
Retrieving Information from Functions
Keeping Variables from Colliding
Tutorial: A Simple Quiz
Part Two: Getting Started with jQuery
Chapter 4: Introducing jQuery
About JavaScript Libraries
Getting jQuery
Adding jQuery to a Page
Modifying Web Pages: An Overview
Understanding the Document Object Model
Selecting Page Elements: The jQuery Way
Basic Selectors
Advanced Selectors
jQuery Filters.
Understanding jQuery Selections.
Adding Content to a Page
Replacing and Removing Selections
Setting and Reading Tag Attributes
Classes
Reading and Changing CSS Properties
Changing Multiple CSS Properties at Once
Reading, Setting, and Removing HTML Attributes.
Acting on Each Element in a Selection.
Anonymous Functions.
this and $(this)
Automatic Pull Quotes
Overview
Programming
Chapter 5: Action/Reaction: Making Pages Come
Alive with Events
What Are Events?
Mouse Events.
Document/Window Events
Form Events.
Keyboard Events.
Using Events the jQuery Way
Tutorial: Introducing Events
More jQuery Event Concepts.
Waiting for the HTML to Load
jQuery Events
The Event Object
Stopping an Event’s Normal Behavior
Removing Events
Advanced Event Management
Other Ways to Use the bind() Function.
Tutorial: A One-Page FAQ
Overview of the Task
The Programming
Chapter 6: Animations and Effects
jQuery Effects
Basic Showing and Hiding
Fading Elements In and Out
Sliding Elements
Tutorial: Login Slider
The Programming
Animations
Easing
Performing an Action After an Effect Is Completed
Tutorial: Animated Dashboard
The Programming
Part Three: Building Web Page Features
Chapter 7: Improving Your Images
Swapping Images
Changing an Image’s src Attribute
Preloading Images
Rollover Images
Tutorial: Adding Rollover Images
Overview of the Task
The Programming.
Tutorial: Photo Gallery with Effects
Overview of Task
The Programming.
Advanced Gallery with jQuery FancyBox
The Basics
Creating a Gallery of Images
Customizing FancyBox.
Tutorial: FancyBox Photo Gallery
Chapter 8: Improving Navigation
Some Link Basics
Selecting Links with JavaScript
Determining a Link’s Destination
Don’t Follow That Link
Opening External Links in a New Window
Creating New Windows
Window Properties
Opening Pages in a Window on the Page
Tutorial: Opening a Page Within a Page
Basic, Animated Navigation Bar
The HTML
The CSS
The JavaScript
The Tutorial
Chapter 9: Enhancing Web Forms
Understanding Forms
Selecting Form Elements
Getting and Setting the Value of a Form Element
Determining Whether Buttons and Boxes Are Checked
Form Events
Adding Smarts to Your Forms.
Focusing the First Field in a Form
Disabling and Enabling Fields
Hiding and Showing Form Options
Tutorial: Basic Form Enhancements
Focusing a Field
Disabling Form Fields
Hiding Form Fields
Form Validation
jQuery Validation Plug-in
Basic Validation
Advanced Validation
Styling Error Messages
Validation Tutorial
Basic Validation
Advanced Validation
Validating Checkboxes and Radio Buttons
Formatting the Error Messages
Chapter 10: Expanding Your Interface
Organizing Information in Tabbed Panels
The HTML
The CSS
The JavaScript
Tabbed Panels Tutorial.
Adding a Content Slider to Your Site
Using AnythingSlider
AnythingSlider Tutorial
Customizing the Slider Appearance
Customizing the Slider Behavior
Determining the Size and Position of Page Elements
Determining the Height and Width of Elements
Determining the Position of Elements on a Page
Determining a Page’s Scrolling Position.
Adding Tooltips
The HTML
The CSS
The JavaScript
Tooltips Tutorial
Part Four: Ajax: Communication with the Web Server
Chapter 11: Introducing Ajax
What Is Ajax?
Ajax: The Basics
Pieces of the Puzzle
Talking to the Web Server
Ajax the jQuery Way
Using the load() Function
Tutorial: The load() Function
The get() and post() Functions
Formatting Data to Send to the Server
Processing Data from the Server
Handling Errors
Tutorial: Using the get() Function
JSON
Accessing JSON Data
Complex JSON Objects
Chapter 12: Flickr and Google Maps
Introducing JSONP
Adding a Flickr Feed to Your Site
Constructing the URL
Using the $.getJSON() Function
Understanding the Flickr JSON Feed
Tutorial: Adding Flickr Images to Your Site
Adding Google Maps to Your Site
Setting a Location for the Map
Other GoMap Options
Adding Markers
Adding Information Windows to Markers
GoMap Tutorial
Part Five: Tips, Tricks, and Troubleshooting
Chapter 13: Getting the Most from jQuery
Useful jQuery Tips and Information
$() Is the Same as jQuery()
Saving Selections Into Variables
Adding Content as Few Times as Possible
Optimizing Your Selectors
Using the jQuery Docs
Reading a Page on the jQuery Docs Site
Traversing the DOM
More Functions For Manipulating HTML
Advanced Event Handling
Chapter 14: Going Further with JavaScript.
Working with Strings
Determining the Length of a String
Changing the Case of a String
Searching a String: indexOf() Technique
Extracting Part of a String with slice()
Finding Patterns in Strings
Creating and Using a Basic Regular Expression
Building a Regular Expression
Grouping Parts of a Pattern
Useful Regular Expressions
Matching a Pattern
Replacing Text
Trying Out Regular Expressions
Working with Numbers
Changing a String to a Number
Testing for Numbers
Rounding Numbers
Formatting Currency Values
Creating a Random Number
Dates and Times
Getting the Month
Getting the Day of the Week
Getting the Time
Creating a Date Other Than Today
Putting It All Together
Using External JavaScript Files
Writing More Efficient JavaScript
Putting Preferences in Variables
Ternary Operator
The Switch Statement
Creating Fast-Loading JavaScript
Chapter 15: Troubleshooting and Debugging
Top JavaScript Programming Mistakes
Non-Closed Pairs
Quotation Marks
Using Reserved Words
Single Equals in Conditional Statements
Case-Sensitivity
Incorrect Path to External JavaScript File
Incorrect Paths Within External JavaScript Files
Disappearing Variables and Functions
Debugging with Firebug
Installing and Turning On Firebug
Viewing Errors with Firebug
Using console.log() to Track Script Progress
Tutorial: Using the Firebug Console
More Powerful Debugging
Debugging Tutorial
Appendix A: JavaScript Resources
Index
Please download here 15.62 MB
Debugging JavaScript and CSS Using Firebug
Harman Goei CSCI 571 1/27/13
Please download here PDF | 1.20 MB






0 komentar:
Post a Comment